Have you ever played a carnival game, only to realise that it was impossibly rigged against you and you probably weren’t going to win that enormous panda plushy after all? Well, now it’s your turn to create an impossibly rigged game. Put the reaction speed of your family and friends to the test with this difficult-to-beat invention.
After starting the game by tapping the touch sensor, the buzzer will beep after a random amount of time. The player must then tap the touch sensor as fast as they can, and we measure their reaction speed. If they tap before the beep, they lose. If they tap to slow, they lose. Yeah, this one’s difficult to beat, but as the creator, you’ll be able to cackle down from above as you watch the chaos ensue.
Parts list
What you’ll need
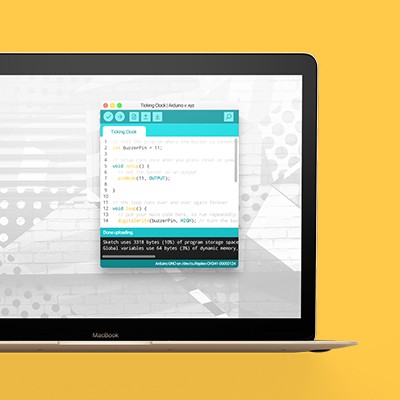
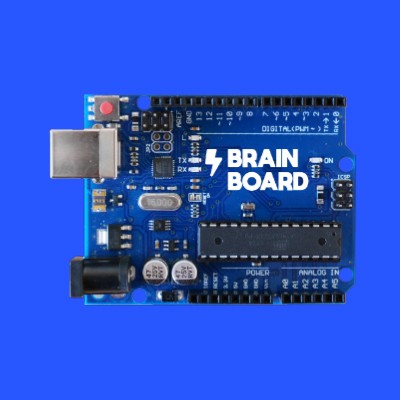
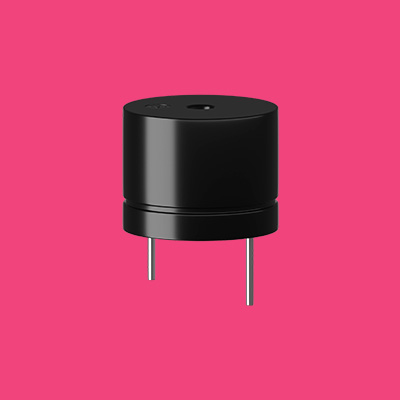
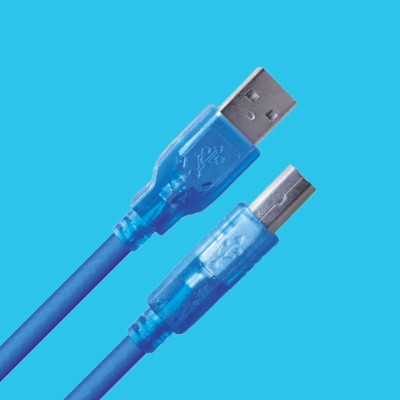
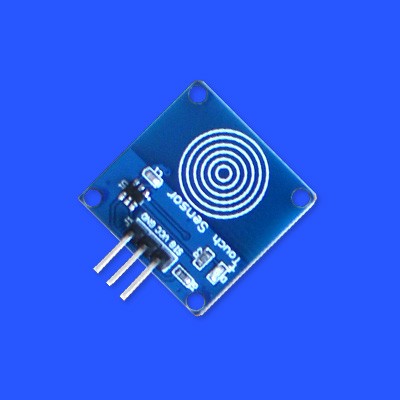
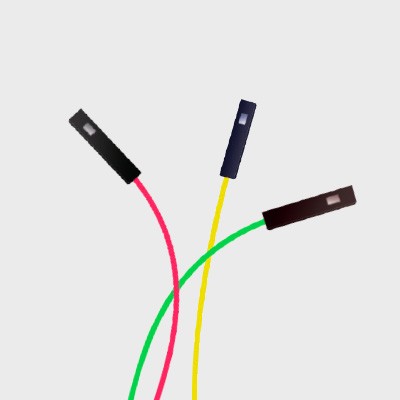
Step 1
Let’s build this
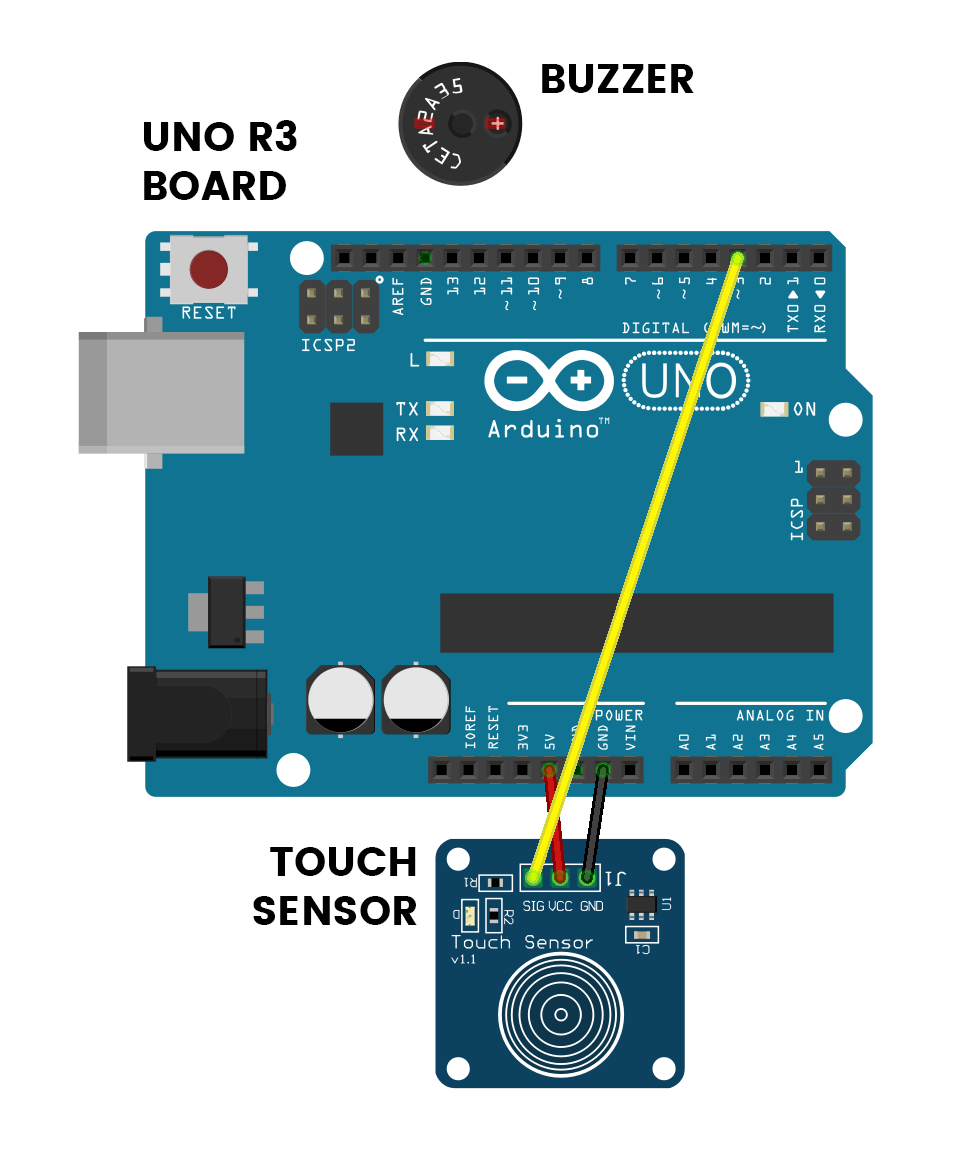
This is the positive leg of the buzzer. It will have a ‘+’ over top and will also be longer than the other leg.
Don’t forget to plug the board into your computer with the supplied USB cable.
The touch sensor is like a button, except that you only have to touch it lightly.
Connections
Buzzer
The +LEG is the longer leg.
Touch Sensor
Use wires to join these.
Step 2
Code some chaos
Don’t forget to select your port, like usual
Copy and paste the sample code
int buzzer = 11;
int button = 3;
bool buttonHandled = true;
bool lastButtonState = LOW;
// The max reaction time (ms) you can have to win
int maxReaction = 500;
/**
* play_round - Starts a new round of the game.
*/
void play_round() {
Serial.println("The game has begun.");
Serial.println("Wait for the beep, then tap the sensor as quickly as you can!");
// Wait a random amount of time to start the game
int timeToWait = random(500, 4000);
delay(timeToWait);
// Disqualify the user if they're already tapping
if (digitalRead(button == HIGH)) {
Serial.println("Well, well, well, we have a cheater.");
Serial.println("You must wait for the beep before you tap the sensor.");
// Exit the game
return;
}
// Make a beep to signal that the game has started
digitalWrite(buzzer, HIGH);
delay(250);
digitalWrite(buzzer, LOW);
// Record the start time
unsigned long startTime = millis();
// Wait for the user to tap the sensor
while (digitalRead(button == LOW)) {
// Do nothing
}
// Calculate the reaction time
unsigned long endTime = millis();
unsigned long reaction = endTime - startTime;
// Print the reaction time
Serial.print("Your reaction time was: ");
Serial.print(String(reaction));
Serial.print("ms\n");
// Determine whether the user won or lost
if (reaction < maxReaction) {
Serial.println("Congratulations! You've WON!");
} else {
Serial.println("Oh no, you've LOST. Better luck next time.");
}
}
/**
* setup - Code here runs once at the start.
*/
void setup() {
// Set up our inputs and outputs
pinMode(buzzer, OUTPUT);
pinMode(button, INPUT);
// Switch on the serial communication
Serial.begin(115200);
}
/*
* loop - Code here runs over and over and over, forever
*/
void loop() {
// Determine if the touch sensor was pressed
if (digitalRead(button) == HIGH && lastButtonState == LOW) {
buttonHandled = true;
}
// Wait for a button press to start a new game
if (buttonHandled == false) {
// Reset the button state
buttonHandled = true;
// Start a new round
play_round();
}
}
Upload the code and open the Serial Port to test it out!
This game uses the Serial Port to send messages to the user. Make sure you’re connected to your computer and have the Serial Port open within the Arduino app to make the best use of this invention!
Step 3
Modify the madness
Change the reaction speed you need to win
On line 8, we created a variable called maxReaction
which sets the maximum reaction speed you can win with. This time is in milliseconds. If the player takes any longer to press the button, then they lose.
// The max reaction time (ms) you can have to win
int maxReaction = 500;
At the moment, we’ve set maxReaction = 500
, which means the user has 500 milliseconds to tap the sensor after they hear the buzzer. By modifying this number, you can make the game easier (use a larger number like 800) or more difficult (use a smaller number like 250).
Change the messages sent to the user
The Brain Board can send messages to the computer over the Serial Port. To write a new messages to the Serial Port, you can use the following code:
Serial.println("Hello, Serial Port!");
Here are some important things to keep in mind:
Serial.println
is the function we are calling. This name is short for “print message over serial, starting a new line once we’re done”.- The message goes in-between the parentheses (curved brackets). Don’t forget to put double quotation marks around your text, otherwise you’ll get an error!
- There’s a semicolon at the end of the line, which signals the end of this instruction.
If you know this structure, you can change any of the messages sent to the user. If you want somewhere to start, try modifying the message on line 14 which lets the user know that the game has started.
Serial.println("The game has begun.");
To modify this message, just change the text string inside the pair of double quotation marks! For example, if we wanted to begin by saying “Are you ready, mortal, to begin your task?”, we’d update this line to:
Serial.println("Are you ready, mortal, to begin your task?");