This invention uses a distance sensor that triggers when someone walks past, setting off an alarm. You can use this to guard your bedroom, give someone a fright, or for actually scaring off real burglars!
Parts list
What you’ll need
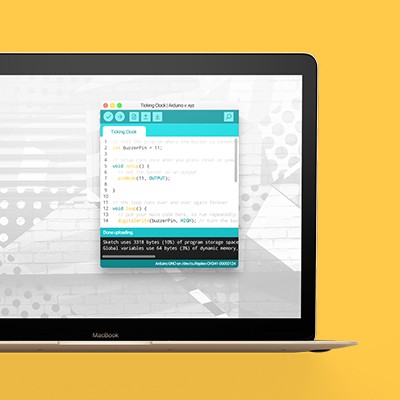
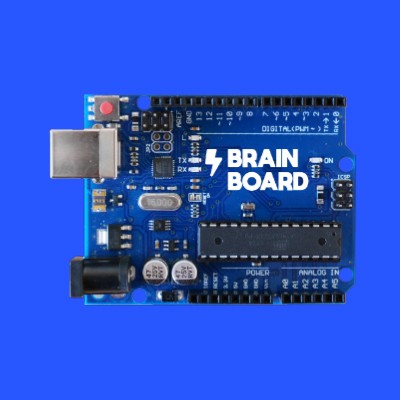
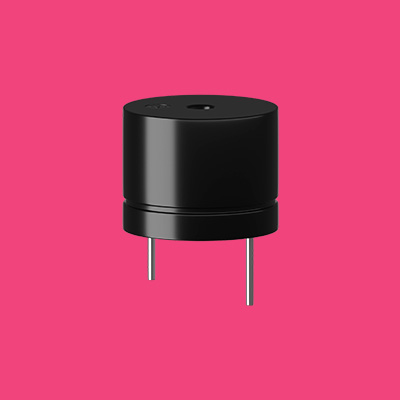
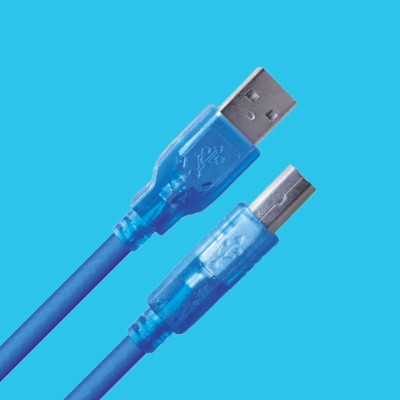
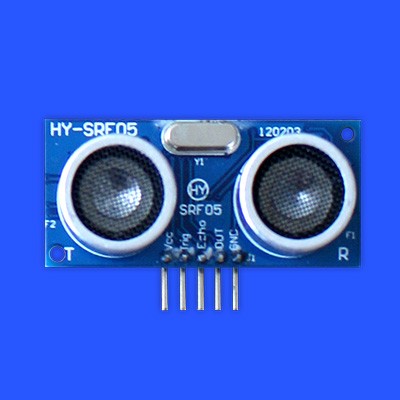
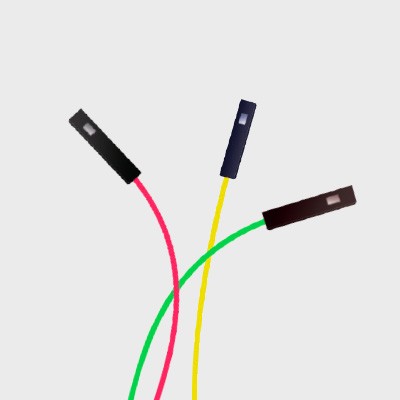
Step 1
Let’s build this
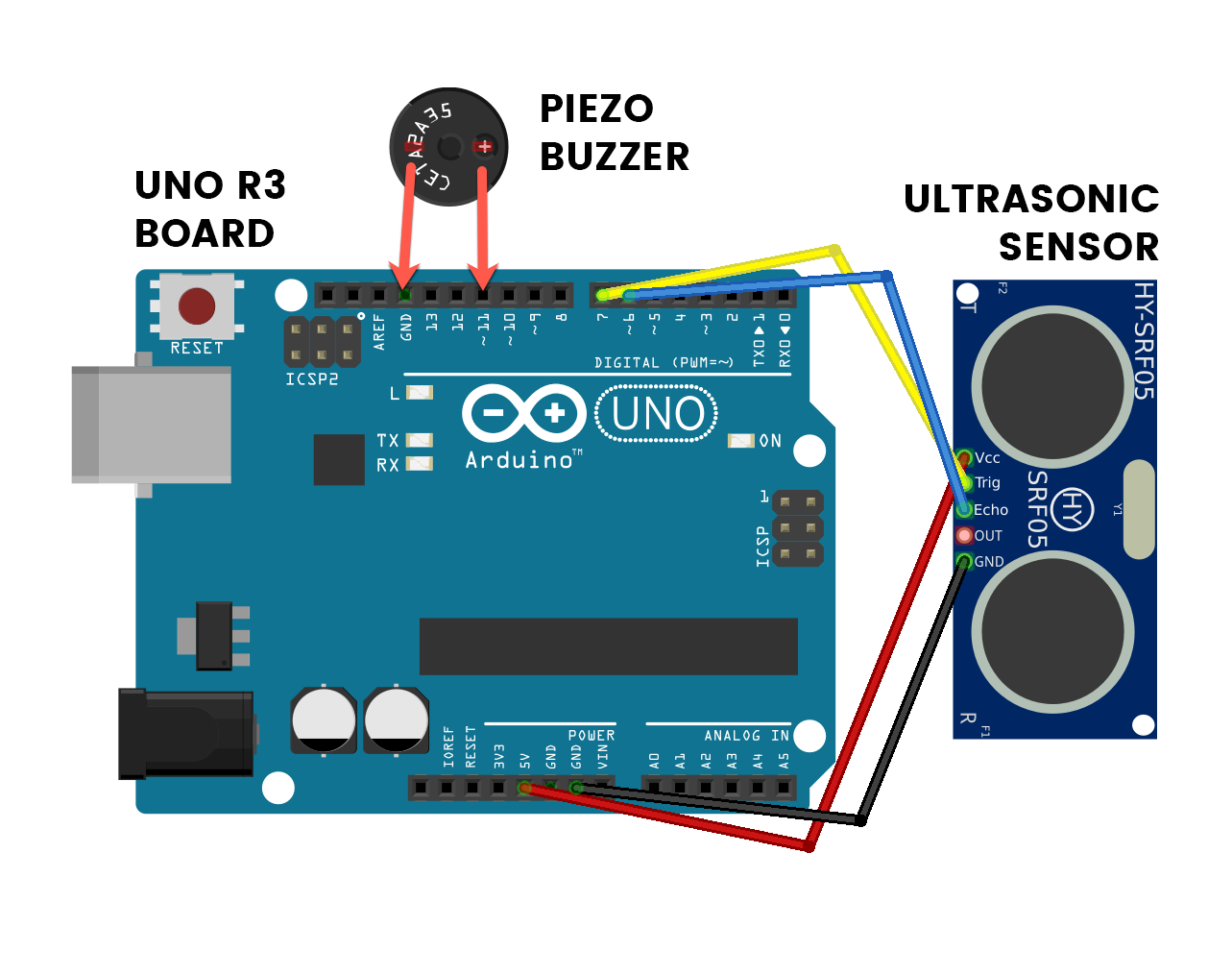
This is the positive leg of the buzzer. It will have a ‘+’ over top and will also be longer than the other leg.
Connections
Buzzer
The +LEG is the longer leg.
Light Sensor
Use wires to join these.
Step 2
Code some chaos
Don’t forget to select your port, like usual
Copy and paste the sample code
int distance() {
int16_t array[3] = {};
for(int count = 0; count < 2; count++){
float duration, distance;
digitalWrite(7, HIGH);
delayMicroseconds(10);
digitalWrite(7, LOW);
duration = pulseIn(6, HIGH) / 2 * 0.0544;
delay(20);
array[count] = duration;
}
int measure = array[0] += array[1] += array[2];
return measure / 3;
}
int repeat = 4;
void setup() {
pinMode(7, OUTPUT);
pinMode(6, INPUT);
pinMode(11, OUTPUT);
Serial.begin(9600);
}
void loop() {
if (distance() < 10) {
for (int count = 0; count < repeat; count++) {
digitalWrite(11, HIGH);
delay(250);
digitalWrite(11, LOW);
delay(250);
}
}
}
Upload the code and test it out
Step 3
Modify the madness
Change how far away the alarm triggers
In the program, the alarm is triggered when someone comes closer than 10cm of the ultrasonic distance sensor. Take a look at line 26 in the code where this happens:
if (distance() < 10) { // If something is closer than 10 cm. . .
If we change the code on line 22, then we make the trigger distance shorter or longer. For example, if we change the number 10 in that line to something like 22, anything that came within 50cm of the sensor would set off the alarm. Here’s what that would look like:
if (distance() < 50) { // If something is closer than 50 cm. . .
Change how long the alarm stays on
Once the alarm has been triggered by someone (or something) coming too close to the ultrasonic distance sensor, we can control exactly how long it stays on for afterwards. In our program, we set the alarm to beep ten times before resetting, ready to be triggered again. Take a look at line 16:
int repeat = 4; // Trigger the alarm for 4 beeps
By changing this code, we can either increase or decrease the number of beeps that the alarm stays on for when triggered. If we wanted it to stay on for 10 beeps, we would use the following code:
int repeat = 10; // Trigger the alarm for 10 beeps
Change the sound of the alarm
We can change the tone – or in other words, the sound – of the beeping using something called the tone() function. Take a look at the code on lines 28-31, which is what we are currently using for our beeps:
analogWrite(buzzer, 255);
delay(500);
analogWrite(buzzer, 0);
delay(500);
To be able to change the tone of the beeps, replace lines 28-31 of your program with the following code:
tone(buzzer, 1000, 500);
delay(1000);
The following code works like this: tone(buzzer, pitch, length). Replace the word pitch with any number you like, and this will control the sound of the beep. Replace the word length with exactly how long you want the beep to go for in milliseconds.
Here’s a different beeping sound that you can try out on your project:
tone(buzzer, 1000, 500);
delay(1000);