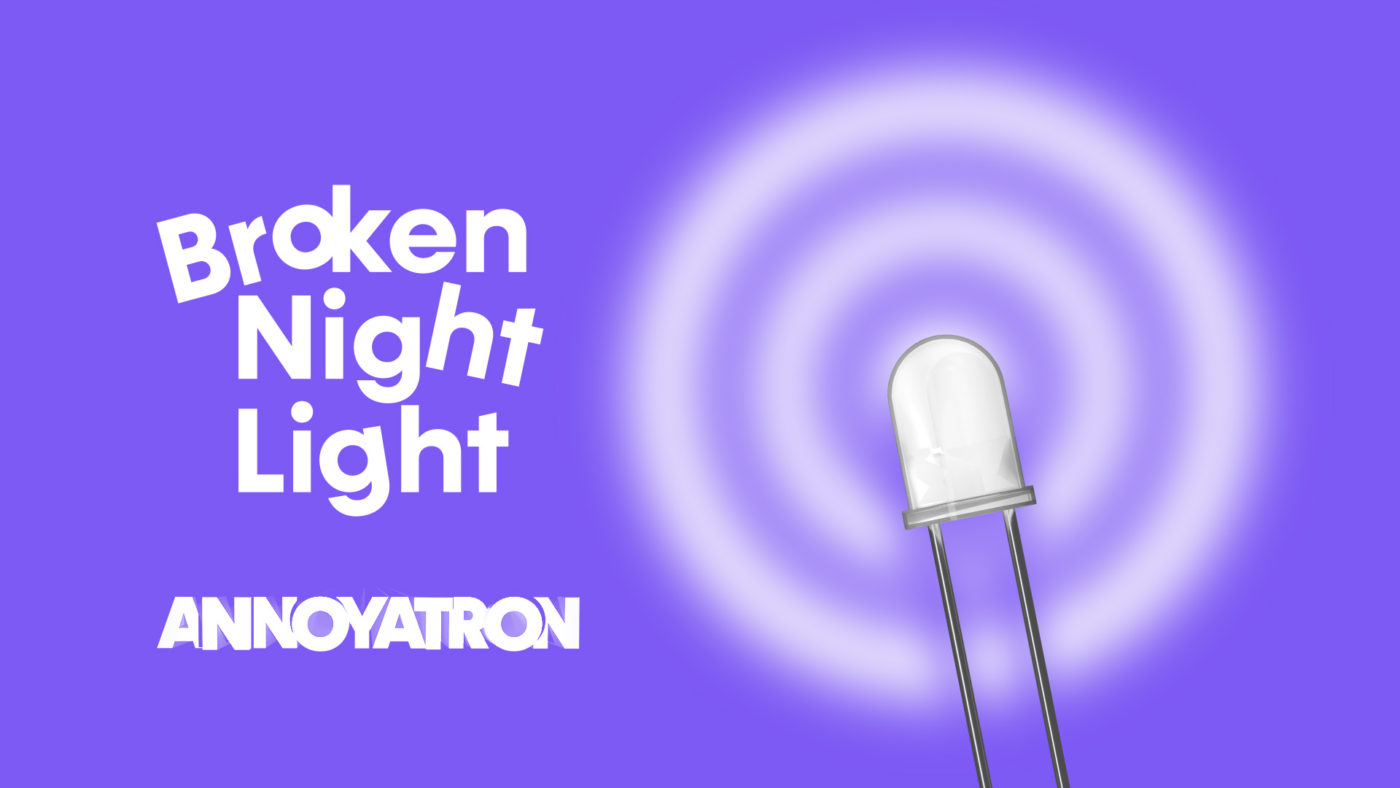
Invention #3
Broken Night Light
Build and code a nightlight that automatically turns off whenever someone walks past! This invention will lead to lots of frustration as people try to navigate the darkness and bump into stuff on the way.
Parts list
What you’ll need
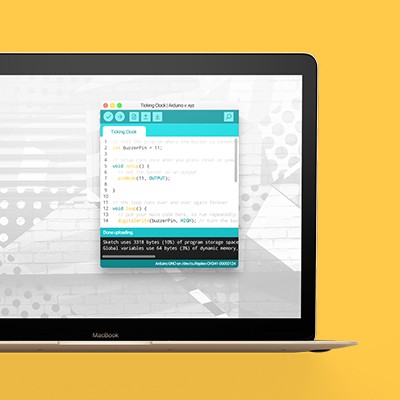
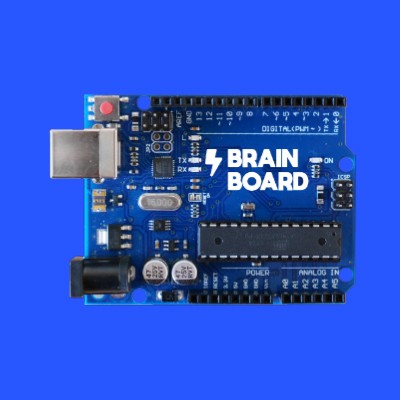
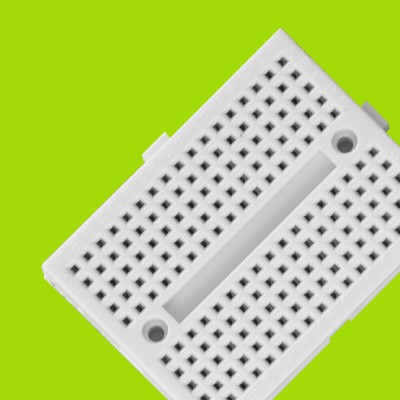
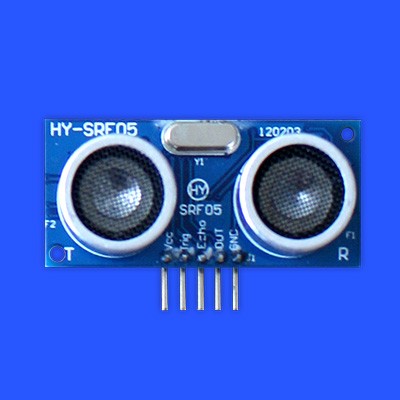
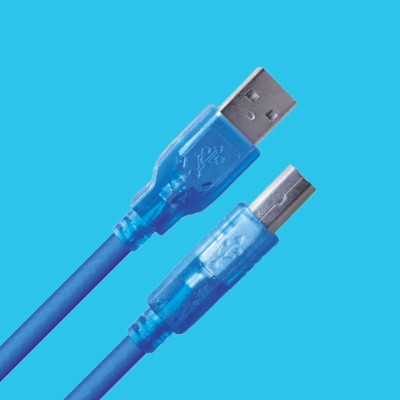
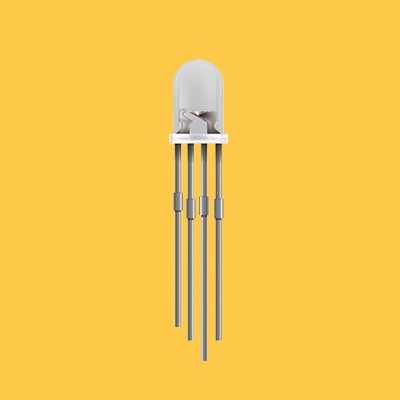
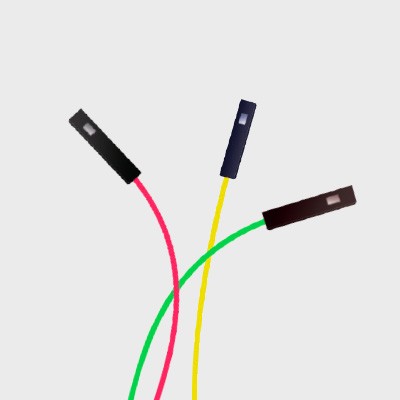
Step 1
Let’s build this

Connections
Distance Sensor
Looks like a pair of eyes 👀
RGB LED
Use wires to join these.
The -LEG is the LONGEST.
Step 2
Code some chaos!
Don’t forget to select your port, as usual.
Copy and paste the sample code
int triggerDistance = 20;
int confusionTime = 2;
int trigPin = 7;
int echoPin = 6;
int redPin = 9; // Where the 'red' LED pin is connected
int greenPin = 10; // Where the 'red' LED pin is connected
int bluePin = 11; // Where the 'red' LED pin is connected
int i = 0;
void setup() {
Serial.begin(9600); // Let the board talk to the computer
for(int l=redPin; l<=bluePin; l++) { pinMode(l, OUTPUT); }
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
}
void loop() {
long duration, inches, cm;
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(5);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
cm = duration / 29 / 2; // Calculate sensor distance
Serial.print(cm); Serial.print("cm"); Serial.println();
if(cm < triggerDistance) { i++; if(i>3) {
analogWrite(redPin, 0); // Turn the brightness to
analogWrite(greenPin, 0); // 0 for all of our LEDS
analogWrite(bluePin, 0); // (we set them up earlier)
delay(confusionTime * 1000);
i = 0; }
} else {
analogWrite(redPin, 100); // Set the brightness
analogWrite(greenPin, 100); // of the LEDs to the
analogWrite(bluePin, 100); // max (100)
i = 0;
}
delay(50);
}
Upload the code and test it out
Step 3
Modify the madness
Change how far away the light will turn off
In the program, the light turns off if someone comes within 20cm of our ultrasonic sensor. However, unless you are expecting your family members to run straight into the light, you will want to change this so that it triggers from further away. Take a peep at line 1 where the trigger distance for the sensor is set.
int triggerDistance = 20; // The trigger distance in cm
As the line of code above notes, the trigger distance value needs to be in centimetres. If we wanted our sensor to trigger from a metre away, we would need to use the following code, as 1 metre = 100 cm:
int triggerDistance = 100; // The trigger distance in cm
Change how long the light will turn off
In this program, there’s something I call the ‘confusion time’. This is basically how long we want to turn off the night light after someone has walked in front of them. In the demo program, this ‘confusion time’ is set to 2 seconds. This can be found on line 2 of the code.
int confusionTime = 2; // Turn the light off for 2 seconds
Don’t think that one second is enough? Want to cause more confusion? Not a bad idea, really. Let’s change it up a little so that now the light will turn off for 3 and a half seconds when triggered. We will need to use the following code:
int confusionTime = 3.5; // Turn the light off for 3 and a half seconds