Ever DJ’d before? Don’t worry, this invention doesn’t require any DJ-ing experience—we’ll teach you everything, right up from the basics. Pressing the touch sensor will trigger a random sound, meaning you don’t know what you’re going to get each time you slam down to make a beat. Tap away at that sweet sound pad and you’ll be the (anti-)DJ Earscreech in no time!
We’re sure your parents will just love this invention. Or maybe not.
Parts list
What you’ll need
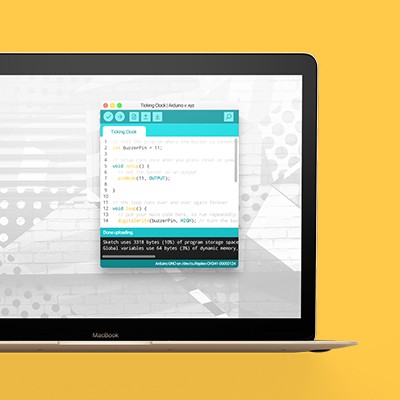
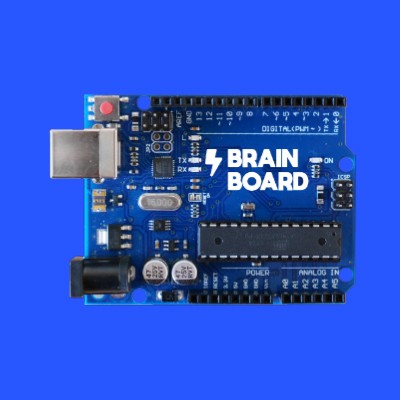
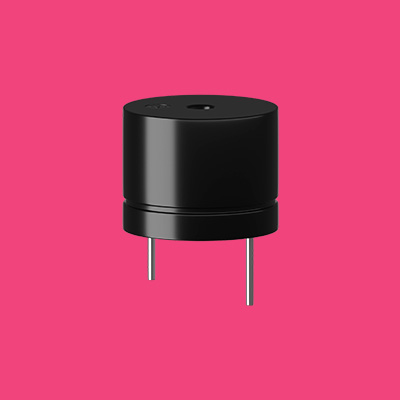
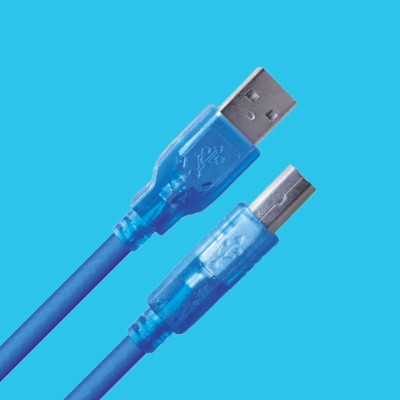
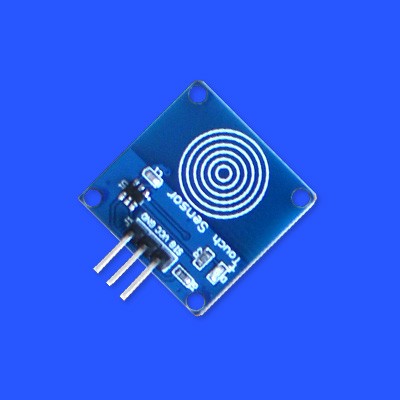
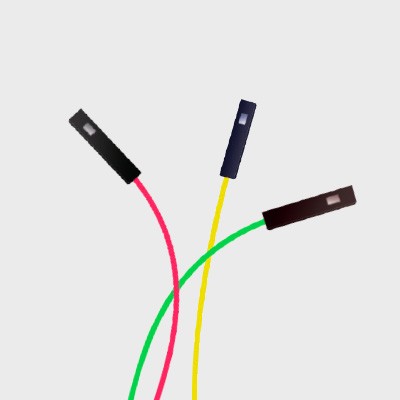
Step 1
Let’s build this
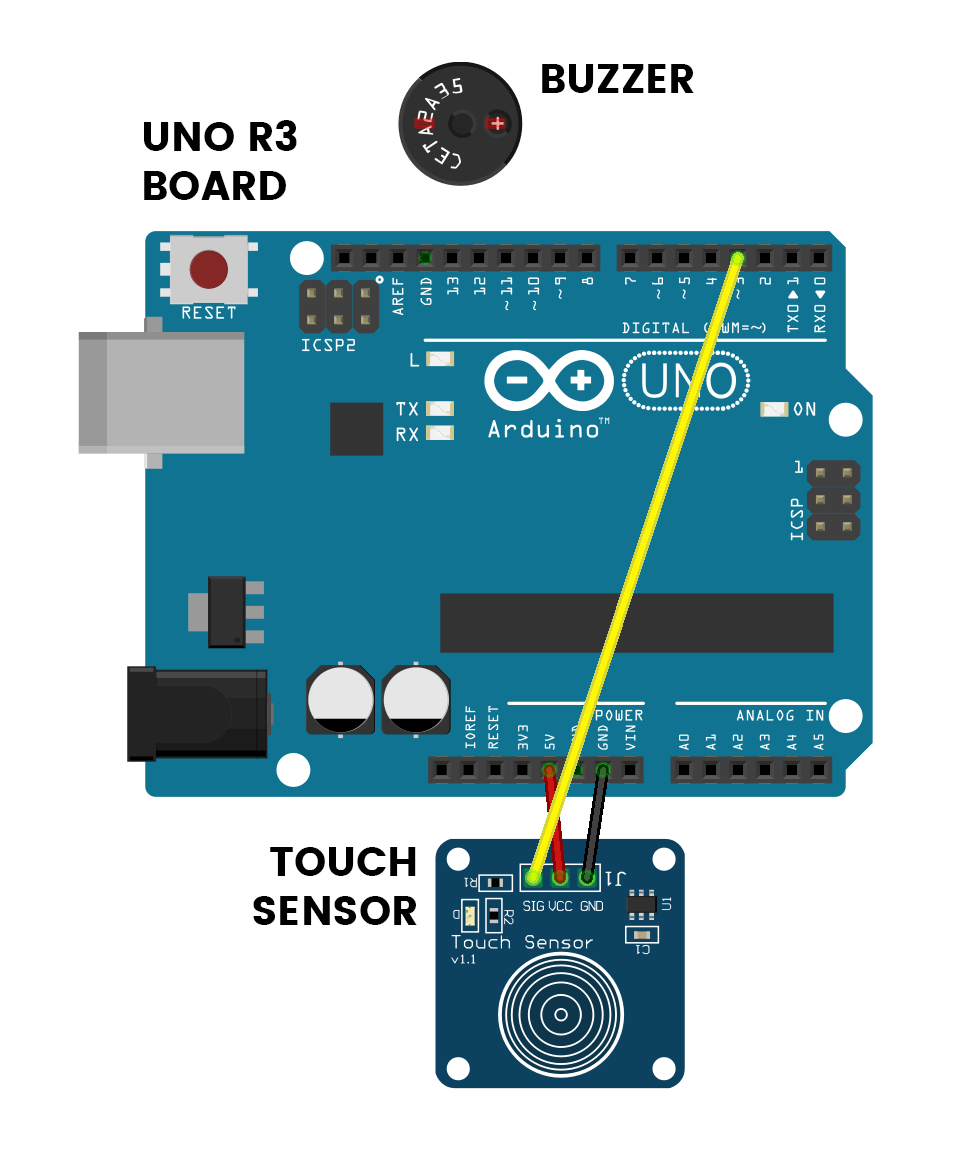
This is the positive leg of the buzzer. It will have a ‘+’ over top and will also be longer than the other leg.
Don’t forget to plug the board into your computer with the supplied USB cable.
The touch sensor is like a button, except that you only have to touch it lightly.
Connections
Buzzer
The +LEG is the longer leg.
Touch Sensor
Use wires to join these.
Step 2
Code some chaos
Don’t forget to select your port, like usual
Copy and paste the sample code
int buzzer = 11;
int button = 3;
bool buttonHandled = true;
bool lastButtonState = LOW;
void setup() {
// Set up our inputs and outputs
pinMode(buzzer, OUTPUT);
pinMode(button, INPUT);
}
void loop() {
// Determine if the touch sensor was pressed
if (digitalRead(button) == HIGH && lastButtonState == LOW) {
buttonHandled = true;
}
if (buttonHandled == false) {
// Generate a random tone
int frequency = random(200, 20000);
int duration = random(50, 750);
// Start the tone on the buzzer
tone(buzzer, frequency, duration);
// Reset the button state
buttonHandled = true;
}
}
Upload the code and test it out
Step 3
Modify the madness
Change the tone frequency and duration
This invention works by playing a random tone through the buzzer every time you press the touch sensor. This random tone comes from 2 numbers: the frequency, which is how high or low pitched the sound is; and the duration, which is how long the tone is played for.
On lines 21-22, we use the random
function to generate random numbers within a range.
// Generate a random tone
int frequency = random(200, 20000);
int duration = random(50, 750);
For example, our frequency is set to a random number between 200 and 20000. See the two numbers in the brackets in random(200, 20000)
? The first number is the minimum, and the second number is the maximum. The random
function will pick a number somewhere in-between.
The same goes for the duration (which is in milliseconds). A random duration is picked between 50 and 750 is selected, which we can tell by looking at the two numbers in the brackets on line 22.