An ultrasonic sensor is a device that measures the distance to an object using inaudible ultrasonic sound waves.
The sensor works by emitting a high-frequency sound wave, waiting for the sound wave to bounce back off an object, and then measuring the time it takes for the echo to return.
We can calculate the distance to the object using the following formula:
\text{distance} = \frac{ \text{speed of sound} \times \text{time taken} } { 2 }
The total distance travelled by the sound wave is just [katex]\text{speed of sound} \times \text{time taken}[/katex], but we divide by 2 because that includes the distance to the object and back—twice our target distance.
The speed of sound is approximately [katex]343 \, \text{m}/\text{s}[/katex], or [katex]0.0343 \, \text{cm} / \mu \text{s}[/katex]. Once we substitute this into our equation, the only variable left is the time taken.
Here’s how we get the time taken for a single pulse to bounce and return:
- Trigger the ultrasonic sensor to send a pulse. We generally do this by emitting a short high pulse (e.g. [katex]10 \, \mu \text{s}[/katex]) to the sensor’s trigger (TRIG) pin.
- Listen to the echo pin of the sensor. This pin will go high for a duration equal to the time taken for the sound wave to travel to the object and back.
- Measure the duration the echo pin stays high. This duration represents the round-trip time of the ultrasonic pulse.
With the round-trip time, you can calculate the distance by simply plugging in to the formula.
To compute the distance value, we need to emit a pulse and calculate the round-trip time, then plug into our distance formula. Let’s start by working through each of our three steps from above to get the round-trip time.
Emit an ultrasonic pulse
Firstly, we need to trigger the ultrasonic sensor to emit the pulse.
// Clear the trigger pin
digitalWrite(triggerPin, LOW);
delayMicroseconds(2);
// Send a 10 microsecond pulse
digitalWrite(triggerPin, HIGH);
delayMicroseconds(10);
digitalWrite(triggerPin, LOW);
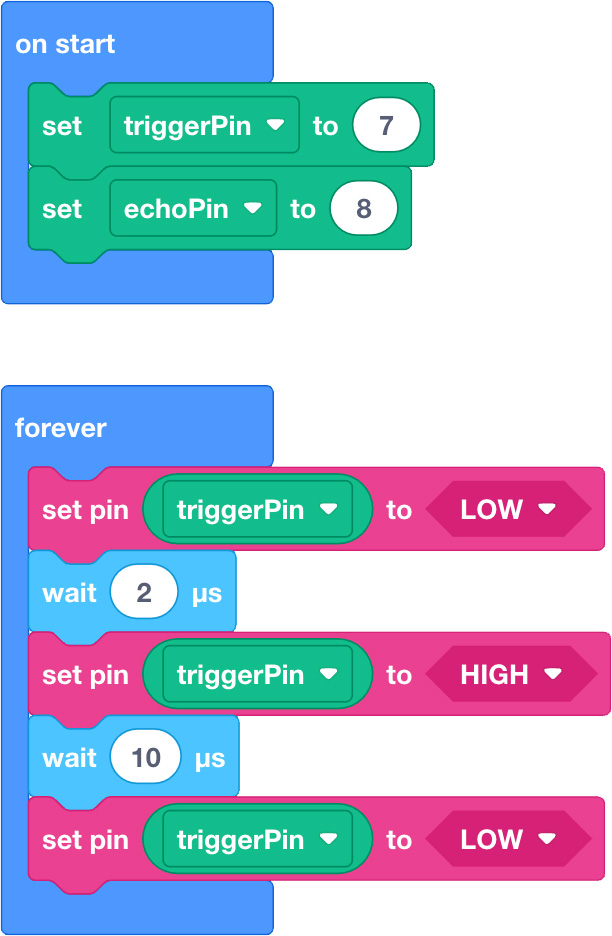
In the snippet, you’ll notice we first pulled the trigger pin LOW
for a brief moment. This ensures a clean start for our pulse and is always good practice to include.
Then, we pull the trigger pin HIGH
for 10 microseconds before then pulling it back to LOW
. This will emit a burst (pulse) of ultrasonic sound waves from the sensor for a duration of 10 microseconds.
Measure the length of the echo
The sensor communicates the round-trip time of the ultrasonic pulse by pulling its echo pin high for that same duration. We can use the special built-in pulseIn
function to wait for the pin to go high, then to measure (in microseconds) how long it stays that way.
// Reach the echo pin
long duration = pulseIn(echoPin, HIGH);
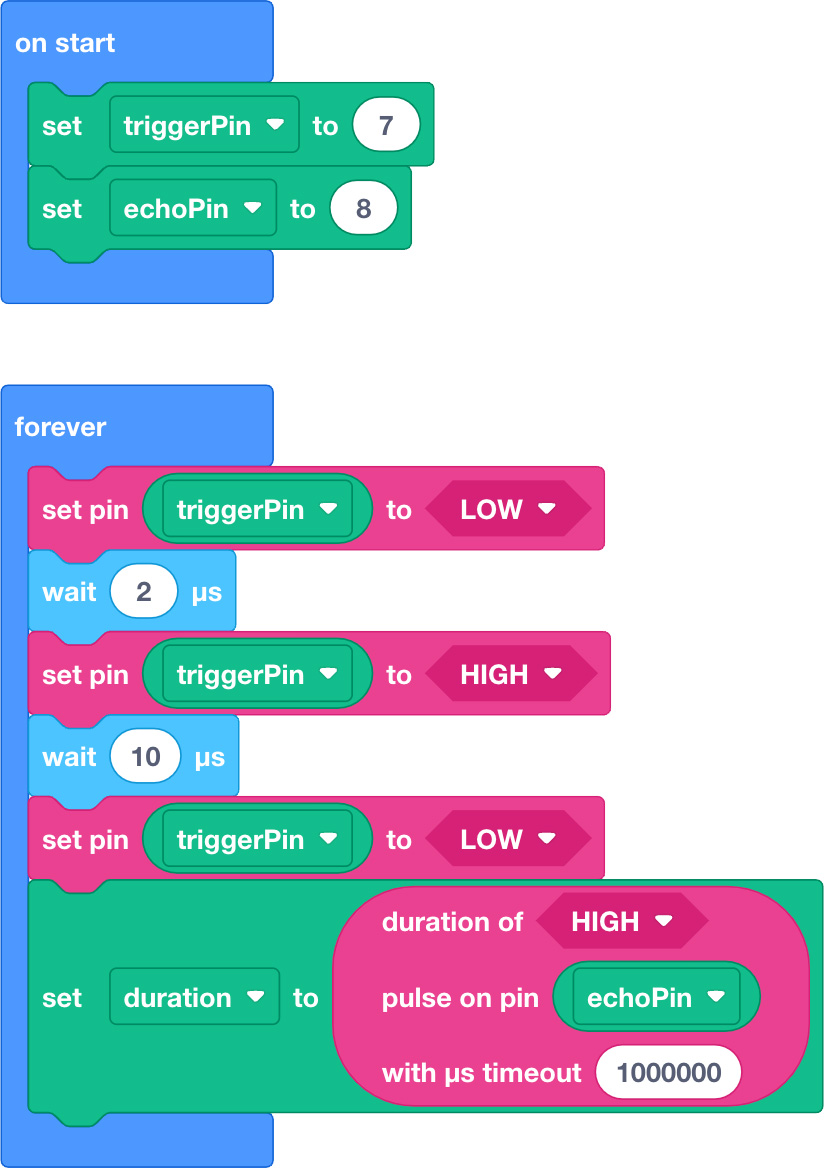
Now, we’ve collected a value for duration
that we can use to compute the final distance.
Compute the distance
Once we have duration
, we can compute the distance via simple substitution. Since our duration
value is in microseconds, we’ll use the sound speed of [katex]0.0343 \, \text{cm} / \mu \text{s}[/katex] for consistency.
// Calculate the distance
int distance = duration * 0.0343 / 2;
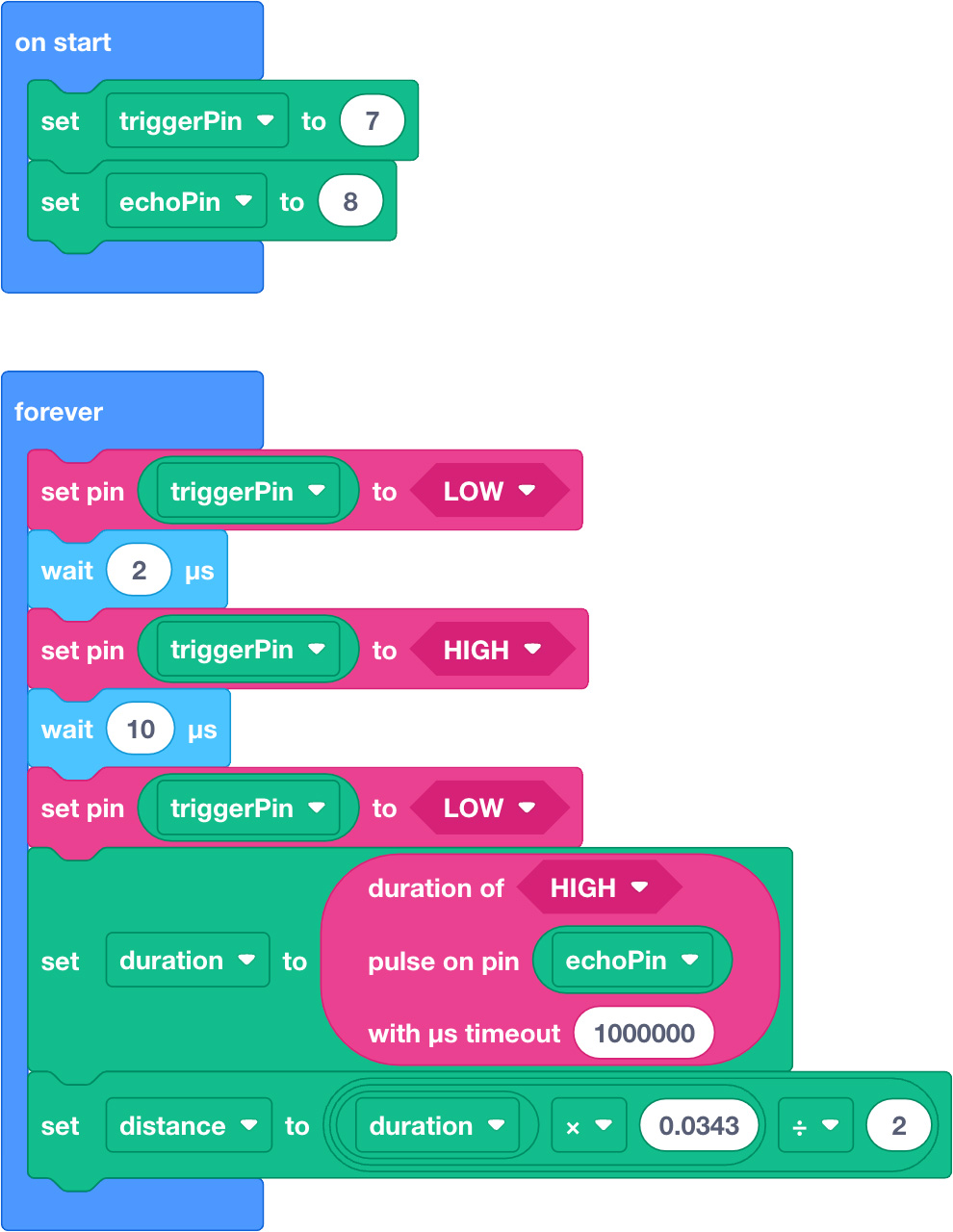
Putting it together
Finally, we can combine all the steps into a single function. This will allow us to measure distance values throughout different parts of our program.
/**
* Measures distance using an ultrasonic sensor.
*/
int measureDistance(int triggerPin, int echoPin) {
// Clear the trigger pin
digitalWrite(triggerPin, LOW);
delayMicroseconds(2);
// Send a 10 microsecond pulse
digitalWrite(triggerPin, HIGH);
delayMicroseconds(10);
digitalWrite(triggerPin, LOW);
// Read the echo pin
long duration = pulseIn(echoPin, HIGH);
// Calculate and return the distance
int distance = duration * 0.0343 / 2;
}
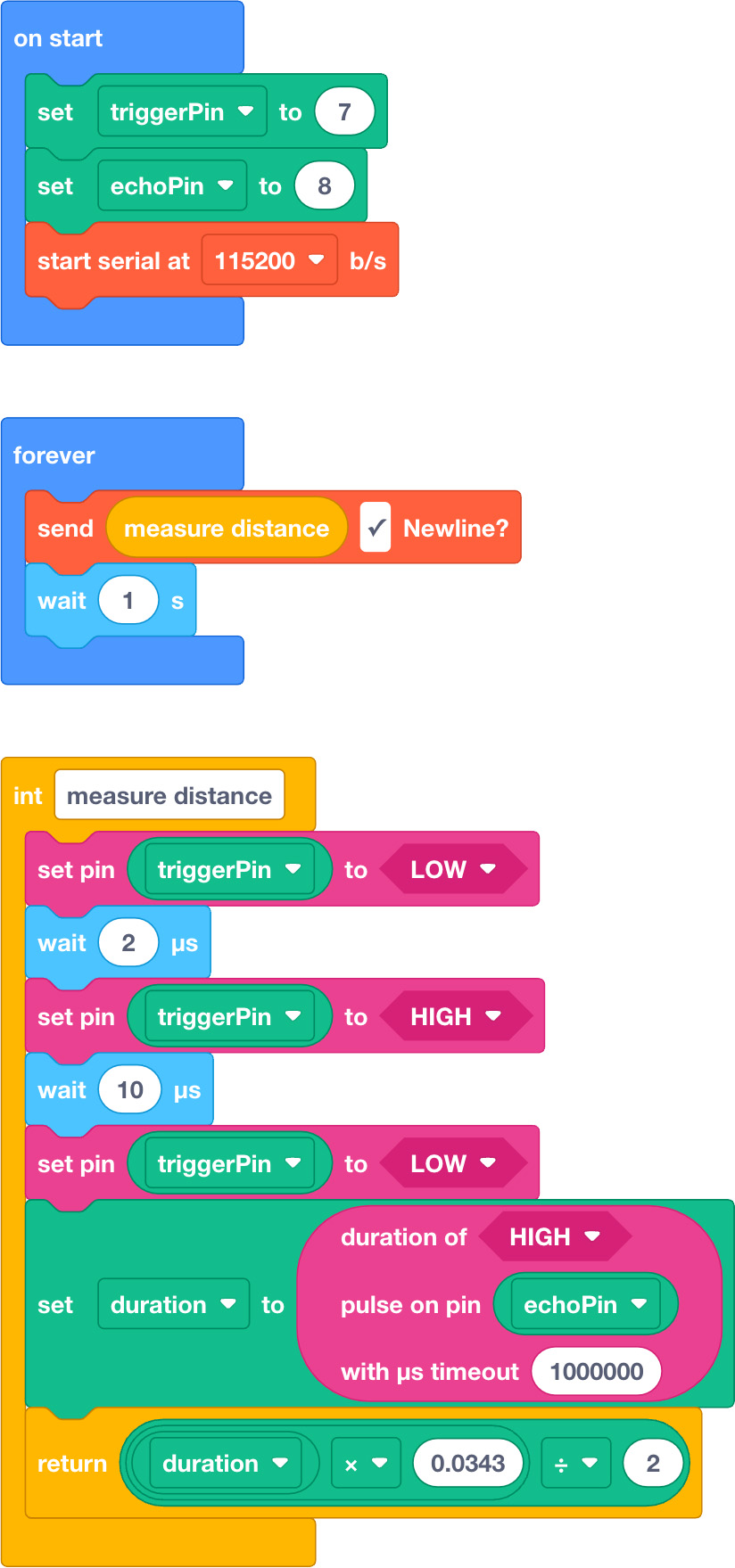